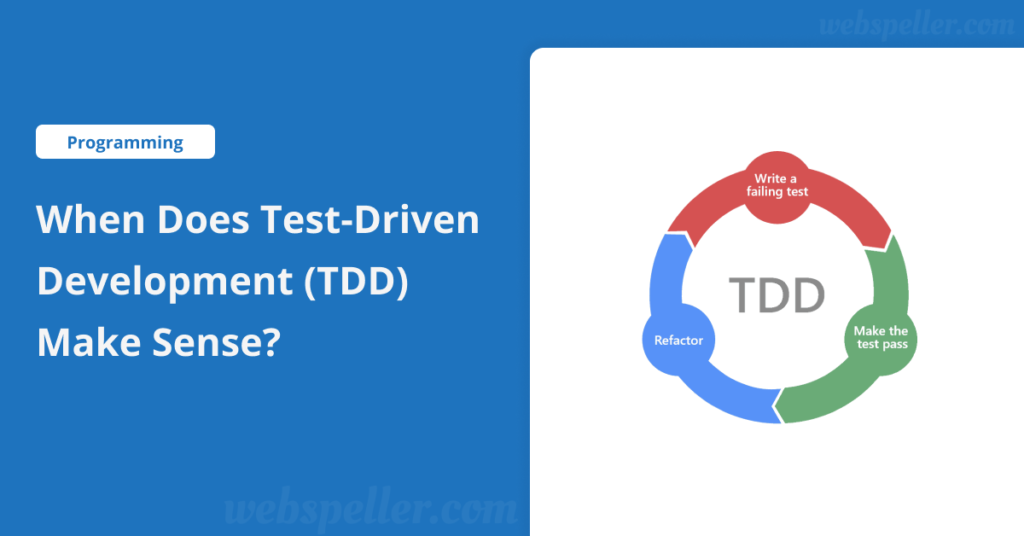
Table of Contents
Introduction
Test-driven development (TDD) is often hailed as a powerful methodology for building robust software. I’ve heard the praises of TDD throughout my career, but for a long time, I couldn’t quite see the benefits. That all changed when I found myself on a project where TDD felt like the perfect fit. It streamlined my development process, sped up my work, and made everything far less prone to errors. In this article, I’ll walk you through when it makes sense to use TDD and why it works best in certain situations.
What is Test-Driven Development (TDD)?
At its core, TDD is a software development process where you write tests before you write the actual code. It’s a cycle of writing a test, writing just enough code to pass that test, and then refactoring the code to improve it. This process originated from Agile principles and Extreme Programming (XP) and is all about letting tests drive the development process.
In simple terms, you write a small test case for a feature, and you don’t write or modify the actual code unless the test fails. It’s a safeguard that helps you avoid duplicated efforts and ensures your code remains clean and functional over time.
TDD in Action: Some Simple Examples
To understand TDD better, let’s take a look at a few real-world examples:
- Calculator Function: Imagine you’re building a simple calculator. With TDD, you would start by writing a test for the “add” function. Once that test passes, you’d move on to writing tests for other functions like “subtract,” “multiply,” and “divide.” You’ll always be guided by the tests to implement only what’s needed.
- User Authentication: Let’s say you’re developing a user login system. With TDD, you’d write a test for the login function before you touch any code. Once the login test passes, you can write new tests for things like registration or password reset, ensuring each feature works as expected without breaking others.
- E-Commerce Website: Building an online store? TDD would involve writing tests for features like product listings, shopping carts, and the checkout process. As you add each feature, your tests ensure the system functions properly—whether it’s adding items to a cart or completing a purchase.
TDD vs. Traditional Testing: What’s the Difference?
- Approach: TDD focuses on writing tests before developing any code. Traditional testing, on the other hand, happens after the code is written.
- Testing Scope: TDD is all about testing individual units of code (like functions), while traditional testing covers larger scopes, like system integration or functional testing.
- Iterative Nature: With TDD, the process is highly iterative. You write a small piece of code, test it, refine it, and repeat. In traditional testing, you often write code first and then test it in bulk later.
- Debugging: TDD allows you to catch issues early, making bugs easier to track down. Traditional testing, done later in the process, may require more effort to trace the root of a problem.
- Documentation: In TDD, your test cases effectively serve as documentation, showing what the code is supposed to do. In traditional testing, you often have more detailed documentation about the entire testing process, environment, and outcomes.
When TDD Isn’t the Best Fit
TDD is powerful, but it’s not a one-size-fits-all solution. Here are some scenarios where TDD might not be the best approach:
- Unclear or Changing Requirements: If the project’s requirements are vague or constantly evolving, writing tests upfront might feel like you’re shooting in the dark. You’ll spend more time rewriting tests than developing actual features. In such cases, it’s often better to experiment and figure things out first.
- Low Domain Logic: If your project mainly revolves around simple tasks like handling input/output (I/O) operations, TDD might be overkill. For example, if you’re building a basic “Hello World” program or writing code that primarily interacts with external systems like databases, the effort required to write tests may outweigh the benefits.
When TDD Works Best
From my own experience, I’ve found that TDD truly shines in the following situations:
- Clear Requirements: When you know exactly what each function needs to do, TDD becomes incredibly effective. You can write tests that accurately represent the desired outcomes, and those tests will guide you through the development process, ensuring everything works as intended.
- Complex Domain Logic: If you’re dealing with a system that has intricate business rules or complex logic, TDD is invaluable. Each test acts as a checkpoint, verifying that your code behaves as expected without breaking any existing functionality. This makes TDD especially helpful in projects where changes can easily introduce regressions.
Why TDD Matters
- Focus on Behavior, Not Implementation: One of the biggest benefits of TDD is that it forces you to focus on what the code should do, rather than how it’s done. This makes it easier to refactor your code later without breaking your tests.
- Long-Term Maintenance: TDD’s biggest advantage is long-term stability. When I revisited a project months after initial development, the existing tests acted like a safety net, allowing me to confidently add new features and improve functionality without worrying about breaking something. That peace of mind is priceless in software development.
A Real-World Example: TDD in a Custom Deck Validation System
In a recent project, I applied TDD while building a custom deck validation Domain Specific Language (DSL) for a card game. The idea was to create a system that lets users define complex deck-building rules in a human-readable format.
The project had two main components that really benefited from a TDD approach:
- RuleValidator: This component checked the user’s input for errors and ensured it followed the right syntax. With TDD, I was able to cover all possible validation scenarios, including edge cases.
- RuleGenerator: This part converted valid user input into TypeScript code, which the system then used to check if a deck followed the rules. Writing tests first made sure that every rule and modifier was correctly implemented.
The TDD process not only made development smoother, but it also helped during refactoring. When I added new features or broke larger functions into smaller ones, the tests caught any potential issues. It was a game-changer for the project.
Conclusion
Test-Driven Development can be a game-changer, but only when used in the right context. It works best when your requirements are clear, the domain logic is complex, and long-term code stability is crucial. However, it might slow you down if you’re still exploring the problem space or dealing with simpler tasks. Knowing when to use TDD and when to take a different approach is key to reaping its full benefits.