Table of Contents
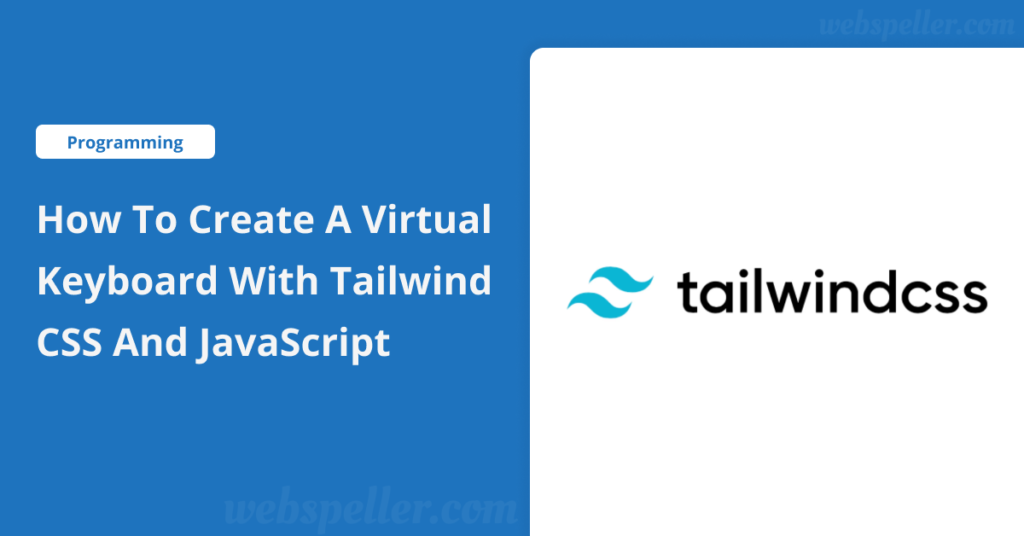
Hey there! Today, I’ll walk you through building a Virtual Keyboard with Tailwind CSS and JavaScript. This project is not only exciting but also an excellent opportunity to enhance your JavaScript skills. Let’s jump right in!
What is a Virtual Keyboard?
A virtual keyboard is software that mimics a physical keyboard, allowing users to input text without physically pressing keys. You’ll often see these on touchscreen devices like smartphones and tablets. Virtual keyboards are super handy for many different tasks, especially where a physical keyboard isn’t available.
Real-World Uses of Virtual Keyboards
Virtual keyboards aren’t just limited to touchscreens; they’re used in many other scenarios:
- Touchscreen Devices: On smartphones and tablets, virtual keyboards are the main method for typing.
- Accessibility: People with physical disabilities can use virtual keyboards to type, whether with a mouse, stylus, or even advanced tools like eye-tracking systems.
- Public Kiosks: Think ATMs or self-checkout machines—virtual keyboards allow input without a traditional keyboard.
- Multilingual Typing: They allow for easy switching between languages without needing to change hardware.
- Security: Some online banking systems use virtual keyboards to prevent hackers from logging keystrokes.
- Gaming: Virtual keyboards can even be useful in gaming, especially in virtual reality (VR) environments where physical keyboards aren’t feasible.
Building the Virtual Keyboard with Tailwind CSS
Now, let’s get into building it! We’re going to use Tailwind CSS for styling and JavaScript to make it interactive.
Step 1: Set Up the Grid
We need more columns to properly arrange the keys on our virtual keyboard. You can extend Tailwind’s grid configuration by adding this to your tailwind.config.js
file:
/** @type {import('tailwindcss').Config} */
module.exports = {
theme: {
gridTemplateColumns: {
1: "repeat(1, minmax(0, 1fr))",
2: "repeat(2, minmax(0, 1fr))",
3: "repeat(3, minmax(0, 1fr))",
4: "repeat(4, minmax(0, 1fr))",
5: "repeat(5, minmax(0, 1fr))",
6: "repeat(6, minmax(0, 1fr))",
7: "repeat(7, minmax(0, 1fr))",
8: "repeat(8, minmax(0, 1fr))",
9: "repeat(9, minmax(0, 1fr))",
10: "repeat(10, minmax(0, 1fr))",
11: "repeat(11, minmax(0, 1fr))",
12: "repeat(12, minmax(0, 1fr))",
13: "repeat(13, minmax(0, 1fr))",
// You can add more if needed
}
}
};
Step 2: Build the HTML Structure
We’ll create a grid layout to arrange the keys, with 13 columns. For simplicity, this example focuses on a small part of the keyboard, but you can expand it as needed.
<div id="virtual-keyboard">
<!-- Output Display -->
<div id="keyboard-output"></div>
<!-- Keyboard Layout -->
<div class="grid gap-1">
<!-- Number Row -->
<div class="grid grid-cols-13 gap-1">
<button class="key">Esc</button>
<button class="key">1</button>
<button class="key">2</button>
<button class="key">3</button>
<button class="key">4</button>
<button class="key">5</button>
<button class="key">6</button>
<button class="key">7</button>
<button class="key">8</button>
<button class="key">9</button>
<button class="key">0</button>
<button class="key">Backspace</button>
</div>
<!-- You can add more rows here -->
</div>
</div>
Step 3: Make the Keyboard Interactive with JavaScript
Now, let’s write the JavaScript to make the keys functional. We’ll listen for click events on the keyboard and display the key presses in the output area.
// Get the keyboard container and output display
const keyboard = document.getElementById("virtual-keyboard");
const output = document.getElementById("keyboard-output");
// Add a click event listener to the keyboard
keyboard.addEventListener("click", function (e) {
if (e.target.classList.contains("key")) {
const key = e.target.textContent;
// Handle different key actions
switch (key) {
case "Backspace":
output.textContent = output.textContent.slice(0, -1);
break;
case "Enter":
output.textContent += "\n";
break;
case "Space":
output.textContent += " ";
break;
case "Tab":
case "Caps":
case "Shift":
case "Ctrl":
case "Alt":
case "Esc":
// These keys won't display output
break;
default:
output.textContent += key;
}
}
});
Step 4: Customize the Layout
Feel free to expand the keyboard layout by adding more rows or customizing the design to fit your needs. Tailwind CSS makes it easy to tweak the styles for each button and the layout as a whole.
Conclusion
And there you have it! A simple virtual keyboard using Tailwind CSS and JavaScript. This project is a great starting point for learning how to handle grid layouts and interactive elements. You can easily extend this basic concept into a fully functional virtual keyboard.
I hope you enjoyed this tutorial! Let me know if you have any questions or suggestions. Happy coding!