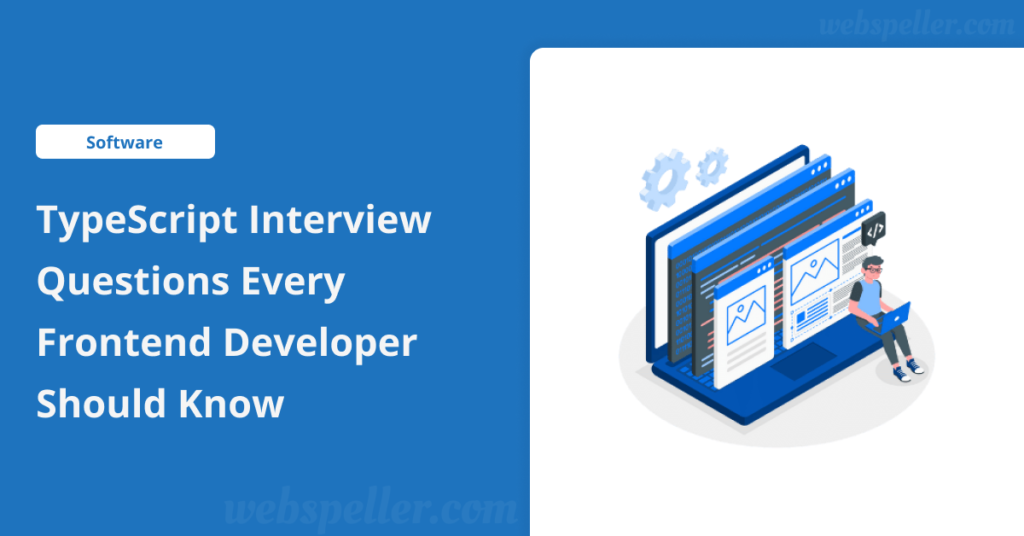
Table of Contents
TypeScript is a critical skill for modern frontend developers. It builds upon JavaScript with strong typing, interfaces, and compile-time error checking. In this article, we will explore 30 commonly asked TypeScript interview questions to help you prepare for a frontend developer role. Mastering these concepts will enhance your chances of acing your interview and securing the job.
Basic TypeScript Syntax & Features
What Are the Key Differences Between TypeScript and JavaScript?
TypeScript is a statically typed superset of JavaScript, which means it extends JavaScript by adding types, interfaces, and compile-time error checking. Unlike JavaScript, which is dynamically typed, TypeScript provides tools for better code scalability and error detection, making it a preferred choice for large projects.
Why Do We Need TypeScript?
TypeScript helps developers catch potential errors during compile-time rather than at runtime. It improves code maintainability with strong typing, enhances the developer experience with better tooling (such as autocompletion), and ensures that your codebase is scalable for larger projects.
What Types Exist in TypeScript?
TypeScript supports a variety of types, including:
- Primitive types like
string
,number
,boolean
,null
,undefined
,symbol
, andbigint
. - Complex types such as
arrays
,tuples
,enums
,any
,unknown
,void
,never
, andobjects
. - Union and intersection types that allow combining multiple types.
What Is the Difference Between type
and interface
?
While both type
and interface
allow you to define custom types, they serve slightly different purposes:
interface
is ideal for defining object shapes and can be extended.type
is more flexible and can be used for unions, intersections, and other complex types.
type CustomType = string | number;
interface User {
id: number;
name: string;
}
What Are Union Types in TypeScript?
Union types allow a variable to hold values of multiple types. They use the |
operator to combine types.
function printId(id: number | string) {
console.log(id);
}
Advanced TypeScript Features
What Are Generics in TypeScript?
Generics enable developers to create reusable components or functions that work with any type while maintaining type safety. Generics make code more flexible and adaptable to various types.
function identity<T>(arg: T): T {
return arg;
}
How Do Generics Constraints Work?
Generics constraints allow you to specify conditions for generic types, ensuring that the provided type meets specific requirements.
function logLength<T extends { length: number }>(arg: T): void {
console.log(arg.length);
}
What Is the Difference Between Partial<T>
and Pick<T, K>
?
Partial<T>
makes all properties ofT
optional.Pick<T, K>
extracts specific properties fromT
.
interface Person {
name: string;
age: number;
}
type PartialPerson = Partial<Person>;
type NameOnly = Pick<Person, 'name'>;
TypeScript and Object-Oriented Programming (OOP)
How Does TypeScript Use Classes?
TypeScript extends the functionality of ES6 classes by adding features such as access modifiers (e.g., public
, private
, protected
) and interfaces. This allows developers to create more robust object-oriented systems with strict type enforcement.
class Person {
private name: string;
constructor(name: string) {
this.name = name;
}
getName(): string {
return this.name;
}
}
What Are Access Modifiers in TypeScript?
Access modifiers control the visibility of class properties and methods:
public
: Accessible everywhere.private
: Accessible only within the class.protected
: Accessible within the class and its subclasses.
What Is the Difference Between an Interface and an Abstract Class?
- An interface is a blueprint for objects, defining a contract with no implementation.
- An abstract class can have both abstract methods (without implementation) and concrete methods (with implementation).
TypeScript in React
How Do You Define Types for Props and State in a React Component?
You can define types for props and state using interfaces or types.
interface Props {
title: string;
}
interface State {
count: number;
}
class MyComponent extends React.Component<Props, State> {
state: State = { count: 0 };
render() {
return <div>{this.props.title}</div>;
}
}
In functional components, props can also be typed using interfaces.
interface Props {
title: string;
}
const MyFunctionalComponent: React.FC<Props> = ({ title }) => {
const [count, setCount] = React.useState<number>(0);
return (
<div>
<h1>{title}</h1>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
What Is the Difference Between JSX.Element and React.ReactNode?
JSX.Element
: Represents a React element returned by a component.React.ReactNode
: Represents anything renderable by React, including elements, strings, numbers, or fragments.
TypeScript in Larger Projects
How Would You Manage Complex Types Across Multiple Files?
In large-scale projects, it’s essential to split types across multiple files. You can use TypeScript’s module system to organize types and import them as needed.
// types.ts
export interface User {
id: number;
name: string;
}
// index.ts
export * from './types';
How Can TypeScript Ensure Type Safety When Working with External APIs?
You can define the expected API response types and use TypeScript’s type system to validate data when consuming external APIs.
interface ApiResponse {
userId: number;
id: number;
title: string;
}
async function fetchPost(id: number): Promise<ApiResponse> {
const response = await fetch(`https://jsonplaceholder.typicode.com/posts/${id}`);
return response.json();
}
Conclusion
Preparing for a TypeScript interview requires a deep understanding of both basic and advanced TypeScript features. By studying the questions and concepts listed here, you’ll be better equipped to demonstrate your proficiency and land the frontend developer role you’re aiming for.