Table of Contents
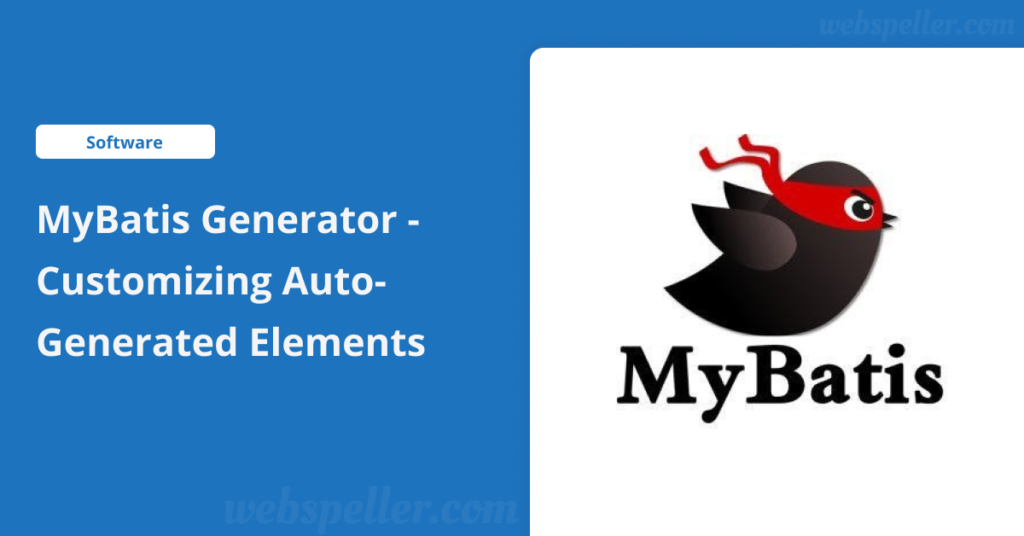
What is MyBatis Generator?
MyBatis Generator (MBG) is a tool used to automatically generate various files that MyBatis requires, based on a database schema. When using MyBatis, SQL typically needs to be handwritten, but writing large amounts of XML manually can lead to mistakes and isn’t particularly efficient. For projects with frequent schema changes, keeping everything updated can also become a hassle. MyBatis Generator solves these issues.
MyBatis Generator is a very flexible and feature-rich tool. In this post, we’ll focus on the merging feature and how to extend the generated files.
What is the Merge Feature?
The merge feature allows MBG to avoid overwriting existing files. Instead, it merges changes, allowing you to repeatedly run the generation process without losing your customizations.
Running MyBatis Generator in Eclipse
We’ll be using Eclipse for this guide, as the merge feature for Java files is only available in the Eclipse plugin version of MBG (version 1.3.1 at the time of writing).
Installing the Eclipse Plugin
For this tutorial, we’ll use Eclipse 3.7.2 with MyBatis Generator 1.3.1.
MyBatis Generator Demo
Creating Tables
To demonstrate, we’ve created two tables, person
and pet
, in a MySQL database named mbgtutorial
.
CREATE TABLE person (
id int,
name varchar(32),
gender varchar(8),
PRIMARY KEY (id)
);
CREATE TABLE pet (
pet_id int,
owner_id int,
pet_name varchar(32),
PRIMARY KEY (pet_id)
);
Creating a Maven Project
We’ll use a Maven project called mbg-tutorial
. Ensure you add the necessary MyBatis dependencies in the pom.xml
file.
Setting Up the MyBatis Generator Configuration File
In Eclipse, go to File → New → Other...
, select MyBatis Generator Configuration File
, and click Next. Save the file as generatorConfig.xml
in the /mbg-tutorial
project directory. Customize the configuration file according to your environment, as shown in this sample:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE generatorConfiguration
PUBLIC "-//mybatis.org//DTD MyBatis Generator Configuration 1.0//EN"
"http://mybatis.org/dtd/mybatis-generator-config_1_0.dtd">
<generatorConfiguration>
<classPathEntry location="/PATH_TO/mysql-connector-java-5.1.19.jar"/>
<context id="context1">
<commentGenerator>
<property name="suppressDate" value="true" />
</commentGenerator>
<jdbcConnection driverClass="com.mysql.jdbc.Driver"
connectionURL="jdbc:mysql://127.0.0.1/mbgtutorial" userId="root" password="" />
<javaModelGenerator targetPackage="net.harawata.domain" targetProject="mbg-tutorial" />
<sqlMapGenerator targetPackage="net.harawata.mapper" targetProject="mbg-tutorial/src/main/resources" />
<javaClientGenerator targetPackage="net.harawata.mapper" targetProject="mbg-tutorial" type="XMLMAPPER" />
<table tableName="person" alias="a_person"/>
<table tableName="pet" />
</context>
</generatorConfiguration>
After configuring, right-click on the generatorConfig.xml
file and select Generate MyBatis/iBATIS Artifacts
.
Extending Generated Files
Next, we’ll extend the generated files to make them more useful. For example, let’s add a list of pets to the Person
class so that whenever we fetch a person, we can also retrieve their pets.
In the Person
class, add the following field and its getter and setter:
private List<Pet> pets;
public List<Pet> getPets() {
return pets;
}
public void setPets(List<Pet> pets) {
this.pets = pets;
}
Then, in the PersonMapper.xml
file, add a new resultMap
that includes this field:
<resultMap id="personWithPetsMap" type="net.harawata.domain.Person" extends="BaseResultMap">
<collection property="pets" resultMap="net.harawata.mapper.PetMapper.BaseResultMap"/>
</resultMap>
Finally, create a SQL query that joins the person
and pet
tables to fetch both:
<select id="selectPersonAndPetsByExample" parameterType="net.harawata.domain.PersonExample" resultMap="personWithPetsMap">
select
<include refid="Base_Column_List" />,
<include refid="net.harawata.mapper.PetMapper.Base_Column_List" />
from person
left join pet on pet.owner_id = id
<if test="_parameter != null">
<include refid="Example_Where_Clause" />
</if>
<if test="orderByClause != null">
order by ${orderByClause}
</if>
</select>
Handling Database Changes
If the database schema changes, for example, by adding an email
column to the person
table:
ALTER TABLE person ADD COLUMN email varchar(255);
You can regenerate the artifacts, and MyBatis Generator will merge the changes while keeping your custom code intact.
Summary
- MyBatis Generator can automatically generate MyBatis-related files from database schemas.
- The Eclipse plugin’s merge feature ensures you can repeatedly run generation without losing custom changes.
- Using MyBatis Generator reduces the effort required to maintain the project when the database schema changes.