Table of Contents
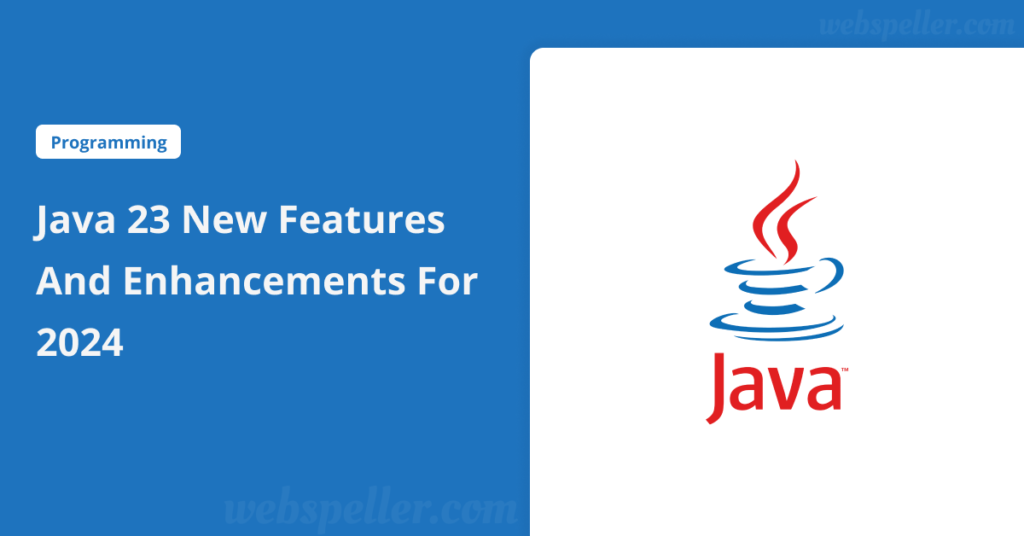
Java 23 New Features, introduced with the September 2024 release, mark a significant milestone in the evolution of the Java language. Building on Java’s tradition of backward compatibility, this version brings a host of performance enhancements, new language constructs, and updated APIs. Designed to cater to both experienced Java developers and newcomers alike, Java 23 is engineered to boost productivity, simplify coding processes, and improve overall performance. Whether you are optimizing legacy applications or developing new ones, these features offer powerful tools to streamline development and create more efficient, scalable software.
In this article, we’ll explore some of the key new features in Java 23, complete with examples to showcase their utility.
Java 23 New Features
1. Primitive Types in Patterns and instanceof
Java 23 enhances the instanceof
operator and switch
expressions, allowing them to work seamlessly with primitive types.
Example:
switch (statusCode) {
case 0 -> "Success";
case 1 -> "Warning";
case int i -> "Error with code: " + i;
}
In this example, the switch
expression can handle both specific constants (e.g., 0
, 1
) and fallback to an int
variable (i
) to process other cases dynamically.
With the release of Java 23 in September 2024, the language has introduced new enhancements like JEP-455, which brings primitive types into patterns, instanceof
, and switch statements. While these features are still in preview, they mark a significant change in how Java handles certain types.
Before Java 23
Before JEP-455, developers faced limitations when working with switch statements and instanceof
for primitive types. For example, using boxed types like Double
in a switch would cause compilation errors. The only compatible types were char
, byte
, short
, int
, or reference types, as specified in the Java Language Specification (JLS).
In Java 21, the switch statement was upgraded to allow patterns, which improved code simplicity. However, these changes still didn’t extend to primitive types like int
, leaving developers to work around limitations.
After Java 23: The New Approach
With Java 23’s preview feature enabled, Long
, Float
, Double
, and Boolean
are now allowed in switch statements, removing previous restrictions. Instanceof
has also been enhanced, making it possible to check primitive types directly.
For example, running:
int i = 42;
if (i instanceof int) {
log("i is an instance of int");
}
now outputs correctly, whereas in earlier versions, this behavior wasn’t supported. Additionally, type casting has been refined to ensure the safety of primitives in patterns. For instance, casting an int
to a byte
now handles potential overflows by enforcing stricter rules on casting.
Conclusion
Switch patterns and instanceof
have evolved with Java 23, making it easier for developers to work with primitive types. This is another leap forward, allowing more flexible and efficient code handling through Project Amber.
2. Virtual Threads (Project Loom)
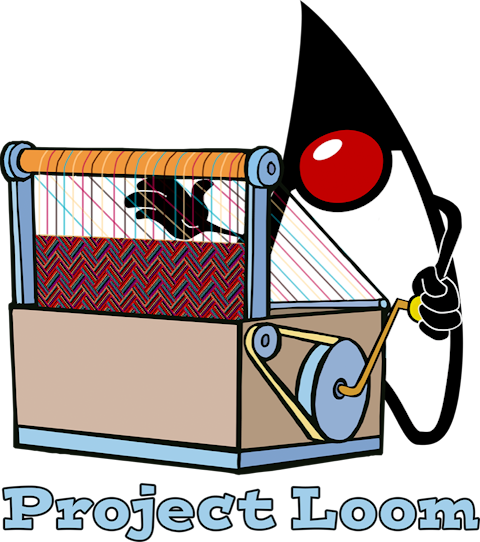
Java 23 takes virtual threads one step further, making it easier to manage high-throughput applications. Virtual threads are lightweight, allowing developers to create thousands of threads without the overhead of traditional platform threads.
Example:
try (var executor = Executors.newVirtualThreadPerTaskExecutor()) {
executor.submit(() -> System.out.println("Task 1"));
executor.submit(() -> System.out.println("Task 2"));
}
Virtual threads can simplify concurrent programming, providing more efficiency when handling a large number of tasks.
Java 19 introduced Project Loom, bringing virtual threads (also known as fibers) to Java, enabling massive scalability. Traditional OS-backed threads are limited in number, but virtual threads allow hundreds of thousands or even millions of threads to be created and managed efficiently. For instance, the code snippet below demonstrates creating 100,000 virtual threads:
try (var executor = Executors.newVirtualThreadPerTaskExecutor()) {
IntStream.range(0, 100_000).forEach(i -> executor.submit(() -> {
Thread.sleep(Duration.ofSeconds(1));
System.out.println(i);
return i;
}));
}
While traditional Java threads struggle with scaling beyond a few thousand threads, virtual threads are much lighter, making them ideal for tasks like handling massive parallel executions, such as performing 100,000 HTTP requests or other I/O-bound operations.
Blocking Calls in Java
A major challenge in multithreaded applications is handling blocking operations, such as reading from an InputStream. Traditionally, when a thread performs an I/O operation, it blocks until the operation is complete, tying up the thread. However, with virtual threads, these blocking calls are internally handled differently by the JVM, making them non-blocking without requiring changes in the code. Here’s an example:
static String getURL(String url) {
try (InputStream in = new URL(url).openStream()) {
return new String(in.readAllBytes());
} catch (IOException e) {
throw new RuntimeException(e);
}
}
When using virtual threads, the JVM delegates the blocking system calls to a more efficient mechanism, such as epoll
on Unix-based systems, allowing non-blocking behavior while maintaining the traditional code structure.
Sockets, Syscalls, and Virtual Threads
When making an HTTP call or performing other socket-based operations, traditional threads block while waiting for data. Operating systems allow these sockets to operate in a non-blocking mode, but it requires developers to write more complex code using libraries like Java NIO. Virtual threads simplify this by abstracting away the non-blocking behavior, allowing developers to write straightforward, synchronous code.
However, file system calls don’t have the same non-blocking capabilities, meaning virtual threads may still delegate these tasks to traditional threads behind the scenes. This gives the illusion of non-blocking file access, but it’s important to understand the underlying limitations.
Virtual Threads and CPU-bound Work
It’s important to note that virtual threads excel in I/O-bound tasks, such as network calls or database interactions, but they don’t offer performance benefits for CPU-bound tasks. Heavy computational tasks won’t see the same improvements in scalability. So, virtual threads won’t help with CPU-intensive tasks like Bitcoin mining or heavy data processing.
Practical Considerations
While Project Loom offers incredible potential for simplifying concurrency, it’s not a magical solution. Real-world applications still have limits, such as socket limits set by the operating system. Virtual threads shine when dealing with I/O tasks like REST calls, database queries, or queue systems, but developers need to be mindful of system constraints and application design.
Conclusion
Project Loom and virtual threads offer a revolutionary way to handle concurrency in Java, especially for I/O-bound tasks. They enable high-throughput applications to scale efficiently without the complexity of traditional asynchronous programming models like RxJava or Project Reactor. However, for developers, understanding the limitations and how virtual threads work under the hood is crucial to achieving optimal performance.
3. String Templates (Preview)
One of the standout features in Java 23 is the introduction of String Templates, which significantly reduces the complexity of string concatenation by allowing expressions to be embedded directly within string literals. This new feature provides a cleaner, more intuitive way to work with dynamic strings, reducing the need for cumbersome concatenation or formatting operations that could easily introduce errors.
For instance, consider the traditional way of concatenating strings in earlier versions of Java:
int apples = 5;
String result = "You have " + apples + " apples.";
System.out.println(result); // Output: You have 5 apples.<br></code>
While this works, it can quickly become cluttered and harder to manage when dealing with multiple variables or complex expressions. The String Templates feature in Java 23 simplifies this by embedding the variable directly into the string:
int apples = 5;
String result = STR."You have \{apples} apples.";
System.out.println(result); // Output: You have 5 apples.<br></code>
This approach is not only cleaner but also reduces the risk of syntax errors, making code easier to read and maintain. The embedded expression \{apples}
allows the variable apples
to be directly interpolated into the string without the need for explicit concatenation.
Complex Expressions and Escaping in String Templates
In addition to simple variable interpolation, String Templates also support more complex expressions, making it easy to embed calculations or function calls within the string. Here’s an example of a more complex use case:
int apples = 5;
int oranges = 3;
String result = STR."You have \{apples + oranges} fruits in total.";
System.out.println(result); // Output: You have 8 fruits in total.<br></code>
In this case, the expression \{apples + oranges}
is evaluated inside the string, allowing for dynamic calculations without additional code outside the string literal.
Java 23 also provides mechanisms for escaping special characters inside string templates, ensuring that expressions do not conflict with normal text:
int apples = 5;
String result = STR."You have \\{apples} apples."; // Escaping the curly braces
System.out.println(result); // Output: You have {apples} apples.
Benefits of String Templates
- Cleaner Syntax: The main advantage of string templates is the elimination of cluttered concatenation. Instead of breaking a string to append variables or expressions, everything stays within a single string literal, improving readability.
- Reduced Error Risk: By embedding expressions directly, string templates help avoid common mistakes, such as missing operators in concatenation or misplacing quotation marks.
- Dynamic String Creation: String templates allow developers to easily create dynamic content without relying on external formatting methods like
String.format()
orprintf()
. This reduces boilerplate code, particularly in cases of multi-line or complex strings. - Improved Maintainability: With simpler syntax and better readability, string templates make it easier to maintain and modify code, especially in larger applications where string manipulation is common.
Real-World Example
String templates shine in scenarios like constructing SQL queries, HTML, or even JSON objects where dynamic content is frequently required. Here’s an example of using a string template to construct a JSON object:
String name = "Alice";
int age = 30;
String json = STR."{
"name": "\{name}",
"age": \{age}
}";
System.out.println(json);
// Output:
// {
// "name": "Alice",
// "age": 30
// }
This approach not only improves readability but also makes it easy to integrate variables directly into JSON structures without resorting to error-prone concatenation.
Conclusion
The String Templates feature in Java 23 marks a significant improvement in how developers work with strings. By allowing expressions to be embedded directly into string literals, it simplifies dynamic string creation, reduces errors, and makes code more maintainable. Whether you’re dealing with basic string concatenation or building complex structures like JSON or SQL queries, String Templates offer a cleaner, more efficient approach to managing strings in Java.
4. Record Patterns (Preview)
Introduced in Java 14, records now support record patterns, allowing developers to destructure and match data classes in a more expressive way.
Example:
record Point(int x, int y) {}
Point point = new Point(3, 4);
if (point instanceof Point(int x, int y)) {
System.out.println("X: " + x + ", Y: " + y);
}
This feature improves readability and simplifies handling complex data structures.
5. Structured Concurrency
Java 23’s structured concurrency simplifies multithreaded programming by managing multiple tasks as a single unit, improving error handling and cancellation.
Example:
try (var scope = new StructuredTaskScope.ShutdownOnFailure()) {
Future<String> result1 = scope.fork(() -> fetchData());
scope.join(); // Wait for all tasks to complete
System.out.println(result1.resultNow());
}
This feature improves the clarity of asynchronous code by grouping related tasks together, which also helps with error management.
6. Foreign Function and Memory API (Project Panama)
Enhancements to the Foreign Function and Memory API enable more efficient and secure interaction between Java and native code. This feature opens up new possibilities for integrating Java with other languages and accessing native memory safely.
Example:
MemorySegment segment = MemorySegment.allocateNative(1024);
try {
MemoryAddress addr = segment.address();
// Interact with native memory
} finally {
segment.close(); // Ensure memory is released
}
The Foreign Function and Memory API streamlines Java’s interaction with native code, offering better performance and security.
Performance Enhancements
1. Garbage Collection
Java 23 brings improvements to Z Garbage Collector (ZGC), making it the default option for better memory management. This reduces pause times and optimizes performance in applications that require efficient memory use.
2. Vector API (Incubator)
The Vector API continues to evolve, offering optimizations for handling parallel computations like machine learning and scientific computing. Developers can take advantage of modern CPU architectures to process data faster.
Example:
var vector = IntVector.fromArray(IntVector.SPECIES_256, new int[]{1, 2, 3, 4}, 0);
IntVector result = vector.add(5);
The Vector API simplifies writing efficient data processing code, especially in performance-critical applications.
Best Practices for Java 23 Development
- Leverage Virtual Threads: Simplify concurrency with Project Loom’s virtual threads to manage thousands of connections with minimal overhead.
- Optimize with Pattern Matching: Use pattern matching in switch statements to enhance code readability and reduce nested conditions.
- Adopt the Vector API: Incorporate the Vector API for handling parallel data processing, improving application performance in areas like AI and data science.
FAQ: Java 23 New Features and Enhancements for 2024
What are the main features introduced in Java 23?
Java 23 includes String Templates, enhanced switch patterns, Project Loom’s virtual threads, and support for primitive types in pattern matching, all aimed at improving productivity and performance.
How do String Templates simplify string handling?
String Templates allow embedding expressions directly into strings using the STR.”…” syntax, making string concatenation more intuitive and reducing errors.
What is the impact of Project Loom in Java 23?
Project Loom introduces virtual threads, making it easier to handle high-concurrency applications by allowing the creation of millions of lightweight threads.
Does Java 23 improve pattern matching?
Yes, Java 23 enhances pattern matching by supporting primitive types in both switch expressions and instanceof
checks, adding more flexibility to code.
Is Java 23 compatible with older versions?
Yes, Java 23 maintains backward compatibility, ensuring that older Java applications continue to function while benefiting from new improvements.
Conclusion
Java 23 introduces a wide range of features and improvements designed to enhance developer productivity, improve performance, and simplify modern development challenges. From virtual threads to string templates, and structured concurrency, this release equips Java developers with powerful tools for building high-performance, maintainable applications.
Java 23 ensures the language remains versatile and adaptable, whether you’re building high-throughput server applications, working with complex data models, or integrating with native code. Developers are encouraged to explore these new features and incorporate them into their projects to stay ahead in an ever-evolving software development landscape.