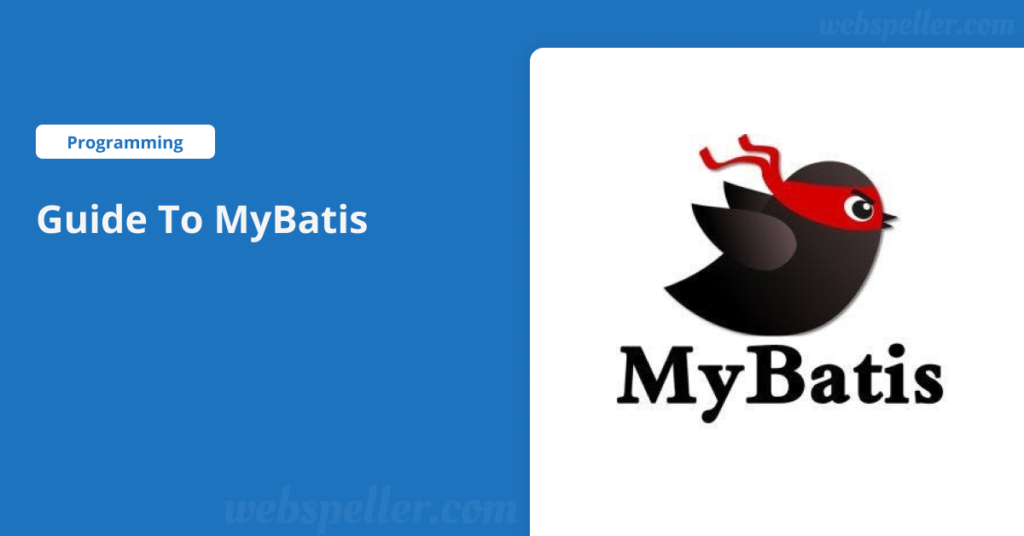
Table of Contents
MyBatis is an open-source database persistence framework that strikes a balance between the low-level JDBC and the higher-level JPA (Java Persistence API). This guide provides an overview of MyBatis and how it differs from other ORM (Object-Relational Mapping) solutions, with practical examples to help you understand its use cases.
What is MyBatis?
MyBatis is a lightweight ORM framework that simplifies the process of mapping database records to Java objects. Unlike JPA, which automatically maps objects to database tables using annotations or XML configurations, MyBatis requires explicit SQL queries for all database operations. This approach gives developers greater control over the SQL while still benefiting from the ORM’s convenience of mapping results to Java objects.
The framework has evolved from its predecessor iBatis and offers powerful features such as dynamic SQL generation, advanced result mapping, transaction management, and caching. With its flexibility, MyBatis is particularly well-suited for complex SQL queries and scenarios where you need fine-tuned control over database interactions.
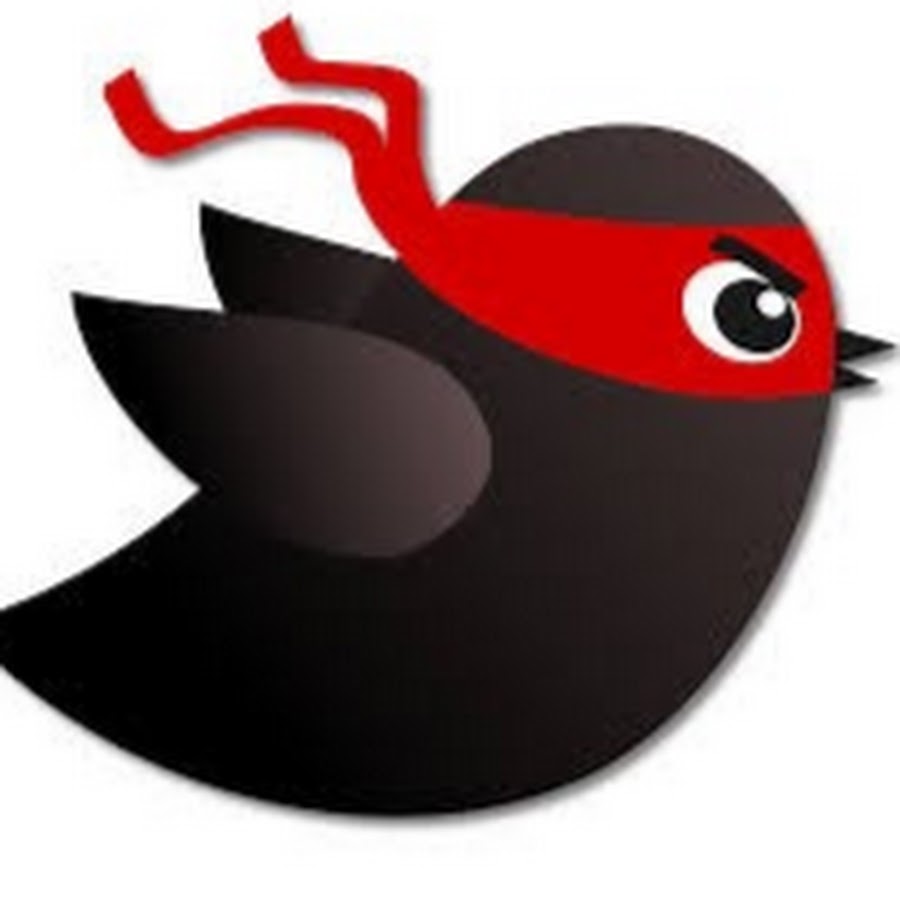
Key Features of MyBatis
- Dynamic SQL Query Generation: MyBatis allows you to create SQL queries that adapt dynamically based on input conditions, making it easy to handle complex logic directly in SQL.
- Advanced Result Mapping: You can map results to Java objects even if the data structures don’t match exactly. This flexibility allows you to use classes that may not be directly modifiable.
- Customizable Mapping: You have complete control over how database columns are mapped to Java object fields.
- Transaction Management: MyBatis supports transaction management, ensuring that database operations are executed reliably.
- Caching: Built-in caching support to improve performance by storing frequently used query results.
- Connection Pooling: MyBatis handles database connection pooling out-of-the-box, reducing the overhead of repeatedly opening and closing database connections.
- Spring Integration: MyBatis integrates seamlessly with popular frameworks like Spring, allowing for easier dependency injection and transaction management.
- MyBatis Generator: A tool that can generate CRUD classes and mappers based on your database schema, saving time on repetitive coding tasks.
MyBatis in Action
Let’s dive into a simple example that demonstrates the usage of MyBatis in a real-world scenario. Imagine you have a relational database with two tables: Session
and Participant
. You need to map these tables into Java classes (Session
and Participant
) and retrieve data from the database in an organized manner.
Sample Database Structure
public class Session {
private Long idFormation;
private String courseName;
private String trainerName;
private Set<Participant> participants = new HashSet<>();
// Getters and Setters...
}
public class Participant {
private Long id;
private String name;
// Getters and Setters...
}
public interface SessionDao {
List<Session> findAll();
}
The Session
class represents a training session, while Participant
stores information about attendees. The SessionDao
interface will handle database operations such as retrieving all sessions.
Using MyBatis for Data Access
To implement the findAll
method using MyBatis, we start by defining our SQL mappings in an XML file:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.SessionDao">
<resultMap id="SessionResultMap" type="com.example.Session">
<id property="idFormation" column="id_formation"/>
<result property="courseName" column="course_name"/>
<result property="trainerName" column="trainer_name"/>
<collection property="participants" resultMap="ParticipantResultMap"/>
</resultMap>
<resultMap id="ParticipantResultMap" type="com.example.Participant">
<id property="id" column="participant_id"/>
<result property="name" column="participant_name"/>
</resultMap>
<select id="findAll" resultMap="SessionResultMap">
SELECT s.id AS id_formation, s.course_name, t.trainer_name, p.id AS participant_id, p.name AS participant_name
FROM sessions s
JOIN participants p ON s.id = p.session_id
JOIN trainers t ON s.trainer_id = t.id
</select>
</mapper>
This XML file specifies how MyBatis should map the query results to Java objects. The resultMap
ensures that the Session
object gets populated with both session details and the list of participants.
Testing the MyBatis Implementation
public class MyBatisSessionDaoTest {
@Test
public void testFindAllSessions() throws Exception {
InputStream inputStream = Resources.getResourceAsStream("mybatis-config.xml");
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
SqlSession sqlSession = sqlSessionFactory.openSession();
SessionDao sessionDao = sqlSession.getMapper(SessionDao.class);
List<Session> sessions = sessionDao.findAll();
// Assert results...
assertEquals(2, sessions.size()); // Expecting 2 sessions
}
}
In the above test case, MyBatis generates the implementation of SessionDao
automatically, thanks to its mapping configurations. You only need to define your SQL queries and let MyBatis take care of the rest.
Conclusion
MyBatis is a powerful and flexible framework that simplifies database interactions while giving you full control over SQL queries. It stands out from other ORM tools by allowing fine-grained customization, making it a preferred choice for developers who need to optimize complex database operations. Whether you are building a small project or a large enterprise application, MyBatis offers the tools needed to streamline your data access layer efficiently.
By following this guide, you can start leveraging MyBatis to enhance your Java applications and manage your relational data with ease.