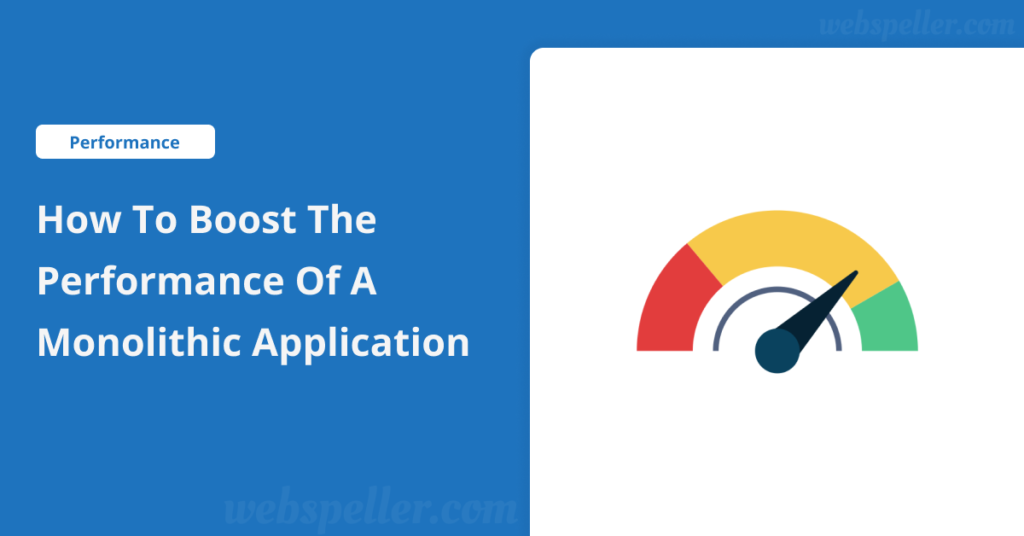
Table of Contents
How to Boost the Performance of a Monolithic Application
Monolithic applications, though often viewed as less scalable compared to microservices, are still widely used in various industries. However, as these applications grow larger and more complex, their performance may begin to lag. To boost the performance of a monolithic application, a complete transition to microservices isn’t always necessary. With the right strategies, you can significantly improve the efficiency of your monolithic architecture without a major overhaul.
In this guide, we will explore several techniques to boost the performance of a monolithic application through database optimization, caching, modularization, scaling, and more.
Optimize Database Queries and Indexing
One of the most common performance bottlenecks in monolithic applications is inefficient database interactions. By optimizing your database queries and indexing strategy, you can significantly improve response times and overall performance.
Strategies to Optimize Database Performance:
- Index Optimization: Ensure that frequently queried fields have proper indexes to improve query speed.
- Query Optimization: Avoid N+1 query issues by implementing techniques such as eager loading or batch fetching.
- Stored Procedures: Move complex business logic to the database with stored procedures to reduce the data transferred between the application and the database.
Example of Query Optimization:
Instead of:
SELECT * FROM orders WHERE customer_id = 123;
Use:
SELECT order_id, order_date FROM orders WHERE customer_id = 123 AND status = 'completed';
Implement Caching Strategies
Caching is a powerful tool to boost the performance of a monolithic application by reducing the load on your database and speeding up response times. Proper caching can minimize redundant operations, especially for data that doesn’t change frequently.
Effective Caching Techniques:
- In-Memory Caching: Use Redis or Memcached to cache frequently accessed data in memory, reducing repeated database queries.
- HTTP Caching: Implement client-side and server-side caching to prevent unnecessary processing of static content.
- Query Result Caching: Cache the results of complex queries that are unlikely to change often, such as product information or other static data.
Example: Implementing Redis Cache in Node.js
import redis from 'redis';
const client = redis.createClient();
const getCachedData = async (key, fetchFunction) => {
return new Promise((resolve, reject) => {
client.get(key, async (err, data) => {
if (err) reject(err);
if (data) {
resolve(JSON.parse(data));
} else {
const freshData = await fetchFunction();
client.setex(key, 3600, JSON.stringify(freshData)); // Cache for 1 hour
resolve(freshData);
}
});
});
};
Reduce Complexity with Modularization
As monolithic applications grow, they tend to accumulate technical debt and become more difficult to maintain. One way to boost the performance of a monolithic application is by modularizing your codebase. Breaking your application into smaller, more manageable pieces can improve performance and maintainability.
Modularization Strategies:
- Service Layer Refactoring: Refactor large services into smaller modules based on functionality. This reduces interdependencies and improves performance.
- Domain-Driven Design (DDD): Structure your application around clear domains, making it easier to isolate and address performance bottlenecks.
- Code Decomposition: Break down large classes or functions into smaller, reusable components.
Horizontal Scaling
While monoliths are often seen as harder to scale, horizontal scaling can still be achieved with the right approach. By adding more instances of your application and distributing the load, you can boost the performance of your monolithic application without transitioning to microservices.
Horizontal Scaling Strategies:
- Load Balancers: Implement a load balancer to distribute traffic across multiple application instances.
- Stateless Services: Ensure that your services are stateless so any instance can handle any request.
- Auto-Scaling: Use cloud solutions like AWS Elastic Beanstalk or Kubernetes to scale your application based on traffic.
Example: Scaling with NGINX
upstream backend {
server backend1.example.com;
server backend2.example.com;
server backend3.example.com;
}
server {
location / {
proxy_pass http://backend;
}
}
Implement Asynchronous Processing
Tasks that don’t need to be executed in real-time can be offloaded to background processes, reducing the load on your monolith. Asynchronous processing is a highly effective way to boost performance by freeing up resources for other tasks.
Asynchronous Processing Strategies:
- Task Queues: Use tools like RabbitMQ or BullMQ to process tasks asynchronously, such as sending emails or generating reports.
- Job Scheduling: Schedule non-urgent tasks to run during off-peak hours.
- Worker Threads: In environments like Node.js, leverage worker threads to handle CPU-intensive operations without blocking the main thread.
Example: Using BullMQ for Asynchronous Processing
import { Queue } from 'bullmq';
const emailQueue = new Queue('emailQueue');
const sendEmail = async (emailData) => {
await emailQueue.add('sendEmailJob', emailData);
};
// Worker to process the job
const emailWorker = new Worker('emailQueue', async job => {
console.log(`Sending email to ${job.data.recipient}`);
});
Optimize I/O Operations
Monolithic applications often face delays due to inefficient I/O operations, such as file handling or external API calls. Improving how your application handles I/O can drastically boost its performance.
I/O Optimization Techniques:
- Batch Processing: Process multiple records or files at once to reduce I/O overhead.
- Stream Data: Use streaming APIs for large files to minimize memory usage.
- Non-blocking I/O: Implement non-blocking I/O for more efficient resource utilization, especially in environments like Node.js.
Leverage Containerization
Containerizing a monolithic application can provide significant performance benefits by improving resource allocation and making scaling easier. Even though the architecture remains monolithic, containerization can help you streamline deployments and optimize resource usage.
Containerization Strategies:
- Dockerize Your Monolith: Containerize your application to standardize environments and improve resource management.
- Kubernetes Orchestration: Use Kubernetes to manage and scale your containerized monolith effectively.
Conclusion
While microservices are often touted as the go-to solution for performance issues, it’s possible to boost the performance of a monolithic application without undergoing a full-scale architectural shift. By focusing on database optimization, caching, modularization, horizontal scaling, and asynchronous processing, you can significantly improve the efficiency and scalability of your monolith. With the right optimizations, monolithic applications can continue to deliver reliable performance for years to come.