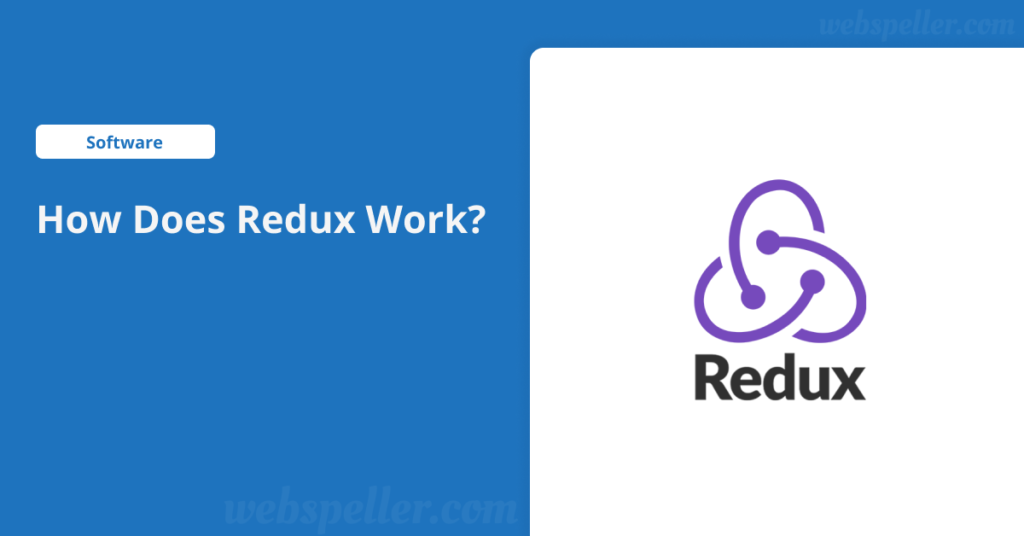
Table of Contents
Here’s a rewritten SEO-friendly article based on the provided French content. The article focuses on the main keyword “How Does Redux Work” and is structured with appropriate headings.
How Does Redux Work?
If you’re involved in web development, you’ve likely come across Redux. But what exactly is Redux, and how does it work? Let’s dive into the core principles and functionality of Redux to demystify its mechanics.
What is Redux?
Redux is a predictable state management library based on the Flux architecture. It allows developers to create a central Store that holds the application’s state. Redux reacts to dispatched actions and notifies subscribers about state changes. Additionally, it supports middleware, enabling pre-processing of actions before they reach the reducers.
1. Managing State
One of the key features of Redux is its state update mechanism, which is defined in reducers. If you’re familiar with Array.prototype.reduce
, you’ll find that your reducer functions have a similar signature.
In simple terms, your state can be represented as follows:
dispatchedActions.reduce(reducer, undefined);
Let’s look at a straightforward example of a reducer:
// Starting from an initial state
const initialState = { counter: 0 };
const counter = (state = initialState, action) => {
switch (action.type) {
case "INCREMENT":
return { counter: state.counter + 1 };
case "DECREMENT":
return { counter: state.counter - 1 };
default:
return state; // Return current state if action is unrecognized
}
};
In this example, the state
parameter defaults to initialState
. When the first action is dispatched, the undefined
accumulator initializes the state to initialState
.
The switch statement returns a new state based on the action type or keeps the current state if the action is not recognized.
2. Subscription Mechanism
To notify interested parties about state updates, Redux incorporates a subscription mechanism, akin to an event emitter. Here’s how it’s set up:
const createStore = reducer => {
let state = reducer(undefined, { type: "@@INIT" });
const subscribers = new Set();
return {
dispatch: action => {
state = reducer(state, action);
subscribers.forEach(func => func());
},
subscribe: func => {
subscribers.add(func);
return () => {
subscribers.delete(func); // Allow unsubscription
};
},
getState: () => state,
};
};
This setup ensures that any time an action is dispatched, all subscribers are notified of the state change.
3. Combining Reducers
To manage larger applications without bloating code, Redux allows the use of multiple reducers. The combineReducers
function enables you to combine multiple reducers into a single reducer that returns a state object structured according to the combined reducers.
const combineReducers = reducers => {
const reducerKeys = Object.keys(reducers);
return (state = {}, action) => {
return reducerKeys.reduce((acc, key) => {
acc[key] = reducers[key](state[key], action);
return acc;
}, {});
};
};
This approach helps maintain clean and manageable code. Here’s how you can use it:
import { users } from "./reducers/user";
import { tweets } from "./reducers/tweets";
const rootReducer = combineReducers({
users,
tweets,
});
const store = createStore(rootReducer);
4. Adding Middleware
Middleware offers great flexibility in Redux. One popular middleware is thunk
, which allows you to pass a function instead of a standard action. This feature is particularly useful for handling asynchronous actions.
Here’s how to implement middleware using the applyMiddleware
function:
const applyMiddleware = (...middlewares) => {
return store => {
const middlewareAPI = {
getState: store.getState,
dispatch: action => dispatch(action),
};
const chain = middlewares.map(middleware => middleware(middlewareAPI));
let dispatch = compose(...chain)(store.dispatch);
return {
...store,
dispatch,
};
};
};
This setup allows you to dispatch actions that can either be regular objects or functions, making Redux highly adaptable to various use cases.
Conclusion
Now that you understand how Redux works, you can approach it with more confidence. Redux offers a structured way to manage application state, enabling you to build scalable and maintainable applications. With its core principles and functionalities, Redux is a powerful tool for any web developer.
By exploring the mechanics of Redux, you can leverage its capabilities to enhance your web development projects effectively.