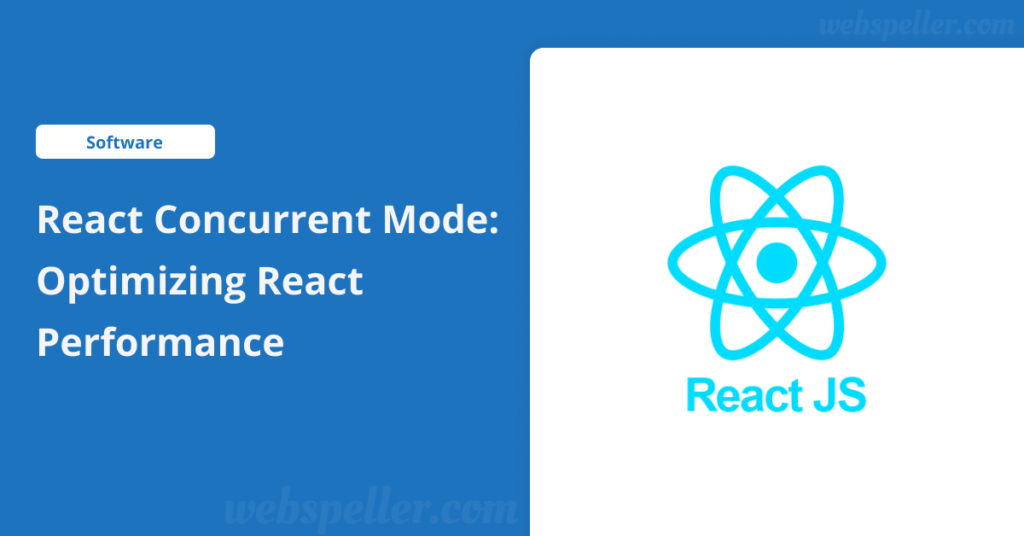
Table of Contents
React Concurrent Mode is a powerful feature that significantly enhances the performance and responsiveness of React applications. By revolutionizing the rendering process, Concurrent Mode allows React to manage multiple tasks simultaneously, ensuring a smoother user experience even during complex updates. In this article, we will explore the key features and benefits of React Concurrent Mode, demonstrating how it can transform your applications.
Key Features of React Concurrent Mode
1. Interruptible Rendering
In traditional React rendering, known as synchronous rendering, tasks are completed one after another. This can lead to delays, especially when dealing with large tasks. With Concurrent Mode, rendering becomes interruptible. React can pause a rendering task to prioritize more critical updates, such as user interactions or animations, before returning to complete the original task later. This flexibility helps maintain a responsive user interface (UI).
2. Time-Slicing
Time-slicing is another vital feature of React Concurrent Mode. This approach breaks up rendering work into smaller chunks, distributing it over time. By doing so, high-priority tasks, such as user inputs or interactions, are given precedence. This prevents the UI from freezing or becoming unresponsive, even during complex updates, ensuring a seamless experience for users.
3. Prioritization of Updates
React Concurrent Mode introduces the ability to prioritize updates based on their importance. High-priority updates, like typing in a text box or clicking buttons, are rendered immediately, while lower-priority tasks, such as rendering large lists, are processed in the background. This smart prioritization allows for a smoother and more interactive user experience.
4. Suspense for Data Fetching
Suspense works hand-in-hand with Concurrent Mode to streamline the handling of asynchronous operations, such as data fetching. With Suspense, components can “wait” for data before rendering. Instead of blocking the entire application, other components can render or display fallback content, like loading spinners, while waiting for the necessary data. This results in a more graceful and efficient loading process.
5. Automatic Batching
Automatic batching is another significant enhancement in Concurrent Mode. Multiple state updates are grouped into a single render cycle, reducing unnecessary re-renders and enhancing overall performance. This batching mechanism ensures that React applications run smoothly, even with frequent state updates.
Benefits of Concurrent Mode
Improved Responsiveness
One of the most notable benefits of React Concurrent Mode is improved responsiveness. The UI remains interactive even when complex rendering tasks are occurring. Users can continue to interact with the application while it fetches data or renders large lists, resulting in a more engaging experience.
Better Performance
By breaking down rendering tasks into smaller chunks, React Concurrent Mode avoids performance bottlenecks. It enables applications to handle large amounts of data or update heavy components without blocking the entire UI. This improvement is crucial for applications that rely on real-time data or require rapid user interactions.
Graceful Data Loading
With the integration of Suspense, React can display loading placeholders while waiting for asynchronous data. This approach allows for a smoother user experience, as users are informed that the application is working on retrieving the necessary information without feeling stuck.
Example of Concurrent Mode with Suspense
To demonstrate how React Concurrent Mode can be utilized, here’s a simple example showcasing the use of Suspense for lazy loading a component:
import React, { Suspense, lazy } from 'react';
const ReactConcurrent = () => {
const LazyComponent = lazy(() => import('../Components/Formmantine'));
return (
<div>
<h1>React Concurrent Mode Example</h1>
{/* Suspense allows loading fallback content while LazyComponent is loading */}
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
export default ReactConcurrent;
Conclusion
In conclusion, React Concurrent Mode is a game-changing feature that enhances the efficiency of React applications. By allowing developers to manage complex updates in the background without compromising the user experience, Concurrent Mode introduces interruptible rendering, time-slicing, and prioritization. These features work together to improve responsiveness and performance, making it an essential tool for any developer looking to optimize their React applications. Embracing React Concurrent Mode will lead to a more dynamic and user-friendly application that can handle the demands of modern web development.