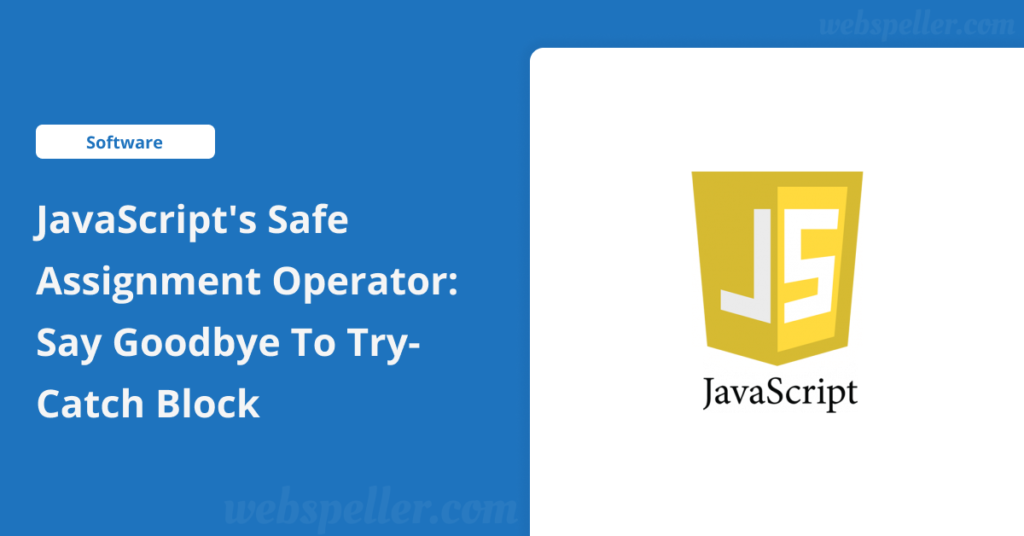
Introduction
JavaScript’s Safe Assignment Operator (?=
) is an exciting proposal that promises to simplify error handling and transform the way developers write cleaner, more efficient code. Traditionally, error handling in JavaScript relied heavily on try-catch blocks, which could make code look messy and hard to maintain. With the introduction of JavaScript’s Safe Assignment, error management becomes more streamlined, helping developers write less cluttered and more maintainable code.
Simplified Error Handling
No More Nested Try-Catch
Try-catch blocks often lead to deeply nested code, making it difficult to read and maintain. The Safe Assignment Operator resolves this by transforming the result of a function into a tuple. If an error occurs, it returns [error, null]
, otherwise, it returns [null, result]
. This approach significantly reduces code nesting and improves readability.
Example:
async function getData() {
const [error, response] ?= await fetch("https://api.example.com/data");
if (error) return handleError(error);
return response;
}
Cleaner, More Linear Code
By using the Safe Assignment Operator, developers can eliminate the clutter caused by multiple try-catch blocks. This operator ensures a more linear flow, making JavaScript code easier to read and understand.
Example:
const [error, data] ?= await someAsyncFunction();
if (error) handle(error);
Consistency Across APIs
One major advantage of JavaScript’s Safe Assignment is its ability to standardize error handling across different APIs. Traditionally, APIs might throw exceptions or return error objects inconsistently. The Safe Assignment Operator ensures uniform error handling, making your code behave consistently no matter the API.
Enhanced Security
Reducing Missed Errors
Missing critical errors is a common risk in traditional error handling, leading to undetected bugs or security vulnerabilities. With the Safe Assignment Operator, the possibility of overlooking important errors is greatly reduced, ensuring that every error is captured and handled appropriately.
Symbol.result: Customizable Error Handling
The Safe Assignment Operator allows objects that implement the Symbol.result
method to define their own error-handling logic. This feature gives developers greater flexibility when managing specific error cases.
Example:
function example() {
return {
[Symbol.result]() {
return [new Error("Error message"), null];
},
};
}
const [error, result] ?= example();
Handling Nested Errors
Recursively Managing Errors
The Safe Assignment Operator can also handle nested errors, making it particularly useful for complex scenarios where errors exist in deeply nested objects.
Example:
const obj = {
[Symbol.result]() {
return [null, { [Symbol.result]: () => [new Error("Nested error"), null] }];
},
};
const [error, data] ?= obj;
Perfect for Promises and Async Functions
JavaScript’s Safe Assignment integrates seamlessly with Promises and async functions. It simplifies asynchronous error handling by embedding error checks directly within async/await code.
Example:
const [error, data] ?= await fetch("https://api.example.com");
Using Statement Integration
The Safe Assignment Operator can be combined with using statements to streamline resource management, making it easier to handle resources and clean up after usage.
Example:
await using [error, resource] ?= getResource();
Prioritizing Error Handling with Safe Assignment
The [error, data] ?=
structure places the error first, emphasizing error handling before data processing. This ensures that developers focus on managing errors before accessing data, reducing the risk of ignoring potential issues.
Example:
const [error, data] ?= someFunction();
Can You Polyfill the Safe Assignment Operator?
Although it’s impossible to polyfill the Safe Assignment Operator directly, you can simulate its behavior using post-processors, allowing compatibility with older JavaScript environments.
Example:
const [error, data] = someFunction[Symbol.result]();
Learning from Other Languages
The concept behind JavaScript’s Safe Assignment is inspired by similar constructs in other programming languages like Go, Rust, and Swift, which have long supported structured error handling. By adopting this method, JavaScript is catching up with modern best practices.
Limitations and Future Improvements
While the Safe Assignment Operator is a promising addition, it’s still a work in progress. One limitation is the lack of a clear term for objects implementing Symbol.result
. Additionally, there’s no new syntax for finally
blocks, though traditional finally
can still be used.
Conclusion
JavaScript’s Safe Assignment Operator (?=
) is set to change how developers handle errors in their code. By reducing the need for verbose try-catch blocks, this proposal brings a cleaner, more secure approach to error management in JavaScript. As this proposal evolves, it could soon become an essential part of every JavaScript developer’s toolkit.