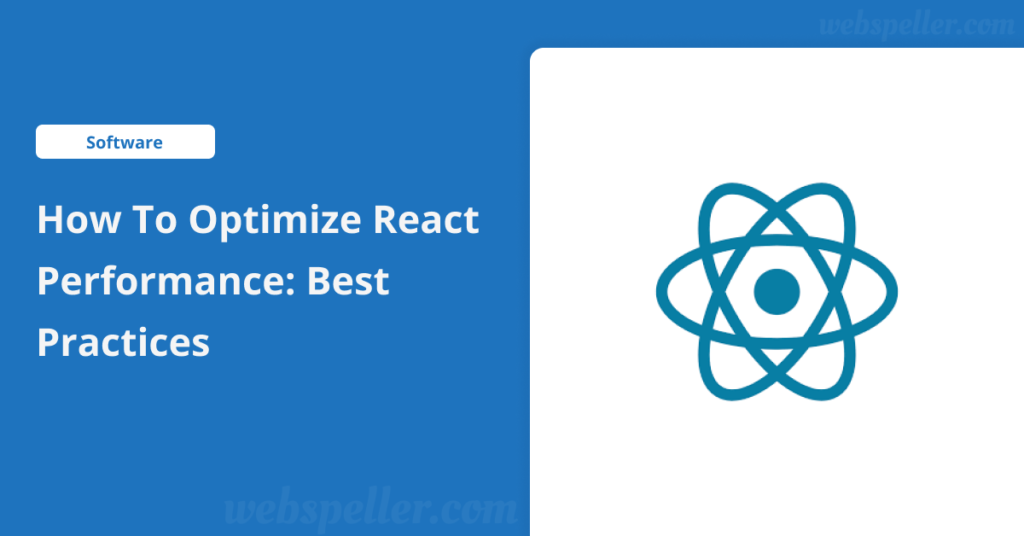
Table of Contents
React is one of the most popular JavaScript libraries for building dynamic user interfaces, thanks to its component-based architecture and efficient rendering mechanisms. However, as your React application scales, optimizing its performance becomes crucial. In this article, we’ll explore key strategies to optimize React performance, helping you build faster and more responsive web apps.
Why Optimize React Performance?
- Improved User Experience: Optimized performance ensures smoother interactions and enhances the overall user experience.
- Faster Load Times: A well-optimized app reduces load times, making it easier for users to access content quickly.
- Increased Responsiveness: Performance improvements lead to more responsive applications, allowing for quicker reactions to user actions.
- Efficient Resource Usage: By optimizing performance, you make better use of system resources, potentially reducing infrastructure costs.
- User Retention: Fast and responsive apps keep users engaged, increasing the likelihood of return visits.
- Project Success: In a competitive market, high performance is key to the success of any project.
Key Strategies to Optimize React Performance
1. Use React.memo()
to Prevent Unnecessary Re-Renders
React.memo()
is a higher-order component that can help optimize the rendering of functional components by memoizing their output. This prevents unnecessary re-renders when the component’s props haven’t changed.
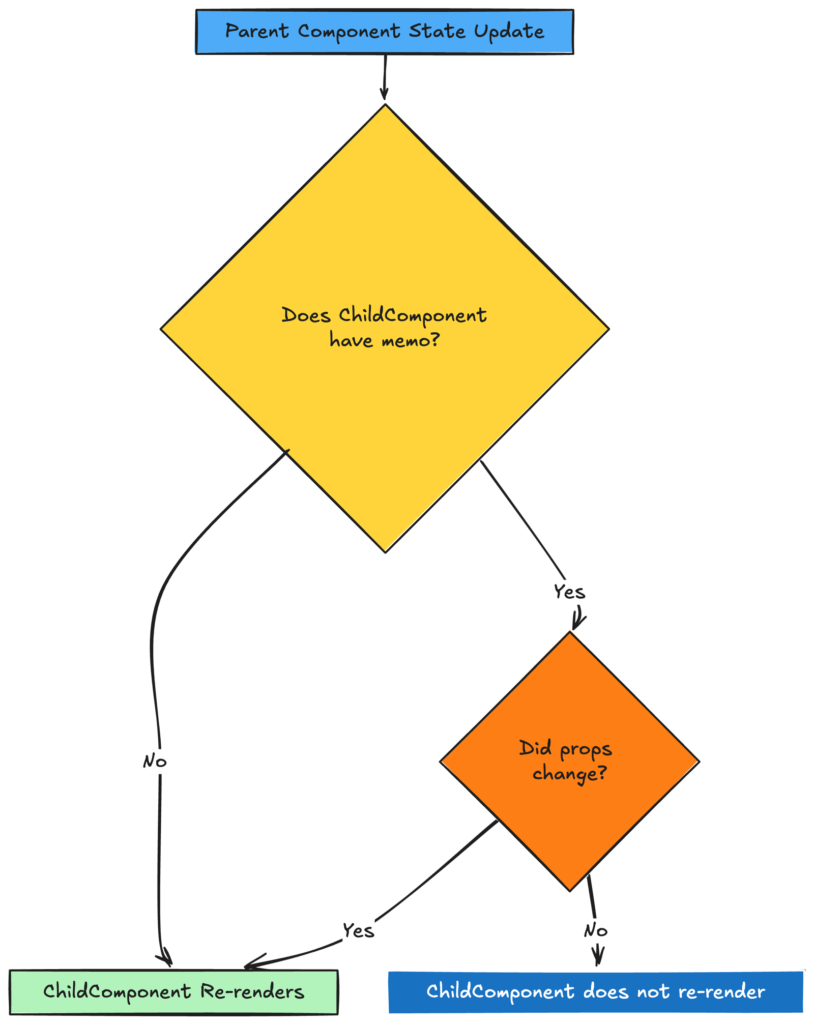
import React, { useState, memo } from 'react';
const CompletedTasks = ({ count }) => {
console.log('Rendering CompletedTasks');
return <div>Completed Tasks: {count}</div>;
};
const MemoizedCompletedTasks = memo(CompletedTasks);
const TaskApp = () => {
const [tasks, setTasks] = useState([
{ id: 1, text: 'Review PR on Artemis', completed: true },
{ id: 2, text: 'Write unit tests on Osmie', completed: false },
{ id: 3, text: 'Peer programming', completed: false },
]);
const toggleTaskCompletion = (taskId) => {
setTasks(tasks.map(task =>
task.id === taskId ? { ...task, completed: !task.completed } : task
));
};
const completedCount = tasks.filter(task => task.completed).length;
return (
<div>
<h1>Task List</h1>
<ul>
{tasks.map(task => (
<li key={task.id}>
<span
style={{ textDecoration: task.completed ? 'line-through' : 'none' }}
onClick={() => toggleTaskCompletion(task.id)}
>
{task.text}
</span>
</li>
))}
</ul>
<MemoizedCompletedTasks count={completedCount} />
</div>
);
};
export default TaskApp;
Why it helps: With React.memo()
, the CompletedTasks
component only re-renders when its props change, avoiding unnecessary updates.
2. Implement Code Splitting with React.lazy()
Code splitting allows you to split your application into smaller bundles, loading only the necessary code when needed. This reduces the initial load time.
import React, { Suspense, lazy } from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
const Home = lazy(() => import('./Home'));
const About = lazy(() => import('./About'));
const App = () => (
<Router>
<Suspense fallback={<div>Loading...</div>}>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</Switch>
</Suspense>
</Router>
);
export default App;
Why it helps: By splitting your code, only the necessary chunks are loaded, speeding up the initial rendering process and improving performance.
3. Use PureComponent
for Class Components
If you’re using class components, switching from Component
to PureComponent
helps optimize rendering. PureComponent
performs a shallow comparison of props and state to decide whether a component needs to re-render.
import React, { PureComponent } from 'react';
class MyComponent extends PureComponent {
render() {
console.log('Rendering MyComponent');
return <div>Hello, {this.props.name}!</div>;
}
}
class App extends PureComponent {
state = { name: 'John' };
changeName = () => {
this.setState({ name: 'John' }); // No real change
};
render() {
return (
<div>
<button onClick={this.changeName}>Change Name</button>
<MyComponent name={this.state.name} />
</div>
);
}
}
export default App;
Why it helps: PureComponent
prevents unnecessary renders, especially when the props and state haven’t changed.
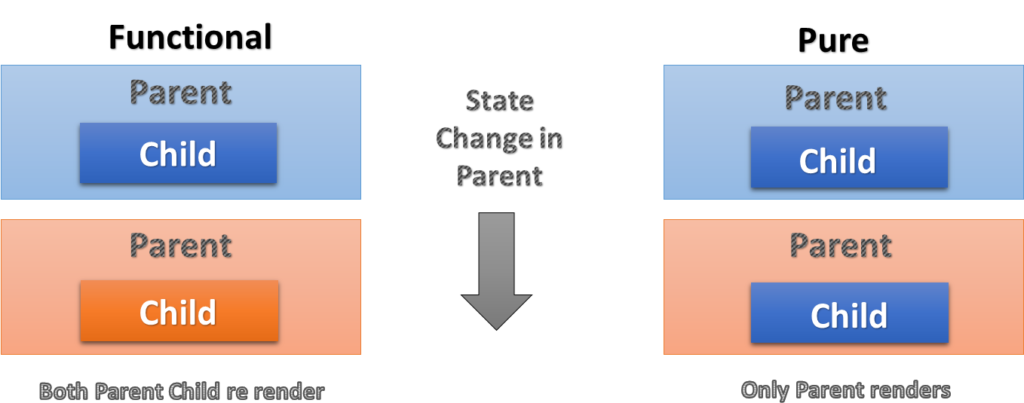
4. Optimize Expensive Renders with useMemo
and useCallback
The useMemo
hook memoizes the result of an expensive calculation, and useCallback
memoizes functions to prevent them from being recreated on each render.
import React, { useState, useMemo, useCallback } from 'react';
const SortedList = ({ list }) => {
const sortedList = useMemo(() => {
console.log('Sorting the list');
return [...list].sort((a, b) => a - b);
}, [list]);
return (
<div>
<h2>Sorted List</h2>
<ul>
{sortedList.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
</div>
);
};
const App = () => {
const [list, setList] = useState([5, 3, 8, 1]);
const [number, setNumber] = useState('');
const addNumber = useCallback(() => {
setList(prevList => [...prevList, parseInt(number)]);
setNumber('');
}, [number]);
return (
<div>
<h1>Number List</h1>
<input
type="text"
value={number}
onChange={e => setNumber(e.target.value)}
placeholder="Enter a number"
/>
<button onClick={addNumber}>Add Number</button>
<SortedList list={list} />
</div>
);
};
export default App;
Why it helps: useMemo
optimizes expensive calculations, and useCallback
prevents unnecessary re-creations of the addNumber
function.
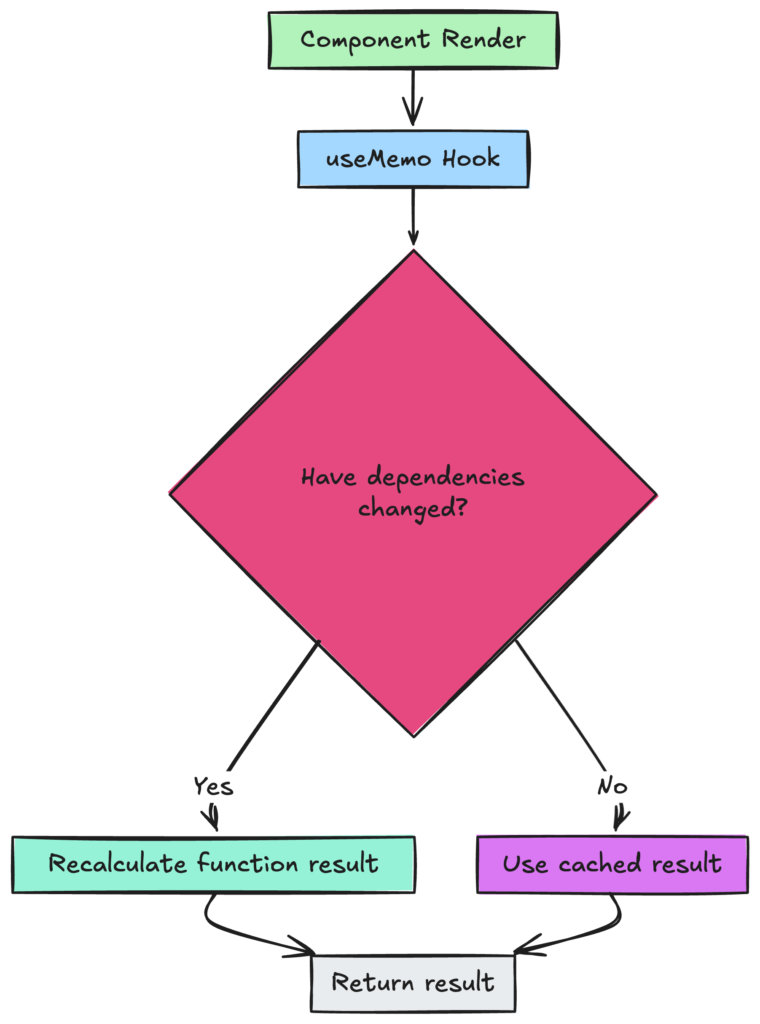
5. Use Reselect for Memoized Selectors in Redux
When working with Redux, Reselect helps memoize derived data, improving performance by preventing unnecessary re-calculations unless the inputs change.
npm install reselect
import { createSelector } from 'reselect';
const getTasks = state => state.tasks;
export const getCompletedTasks = createSelector(
[getTasks],
tasks => tasks.filter(task => task.completed)
);
export const getCompletedTaskCount = createSelector(
[getCompletedTasks],
completedTasks => completedTasks.length
);
Why it helps: Memoized selectors prevent recalculations unless the relevant state changes, improving performance in Redux-based apps.
Conclusion
Optimizing React performance is essential for creating fast, responsive, and user-friendly applications. Techniques like using React.memo()
, code splitting, useMemo()
, useCallback()
, and Reselect with Redux help ensure your application runs smoothly and efficiently. However, always balance performance optimizations with code maintainability and clarity for the best long-term outcomes.
By following these best practices, you can optimize React performance and deliver an outstanding user experience that keeps your users engaged.